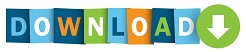

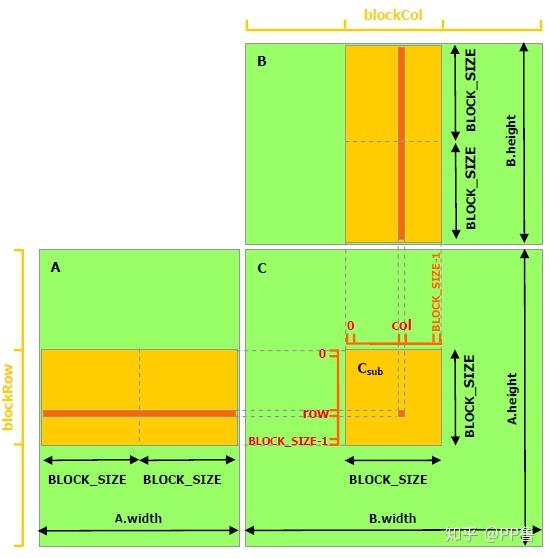
#Pthread c shared memory code#
This might result in a deadlock unless some threads eventually exit or the code Tried to spawn a new thread, but the thread pool is exhausted. However, when you try to run it in a browser or Node.js, you'll see a warning and then the program will hang: Before the thread To compile code using threads with Emscripten, you need to invoke emcc and pass a -pthread parameter, as when compiling the same code with Clang or GCC on other platforms: emcc -pthread example.c -o example.js In this case, there aren't any results so the function takes a NULL as an argument. It accepts the earlier assigned thread handle as well as a pointer to store the result. Pthread_join can be called later at any time to wait for the thread to finish the execution, and get the result returned from the callback. It takes a destination to store a thread handle in, some thread creation attributes (here not passing any, so it's just NULL), the callback to be executed in the new thread (here thread_callback), and an optional argument pointer to pass to that callback in case you want to share some data from the main thread-in this example we're sharing a pointer to a variable arg. Pthread_create will create a background thread.
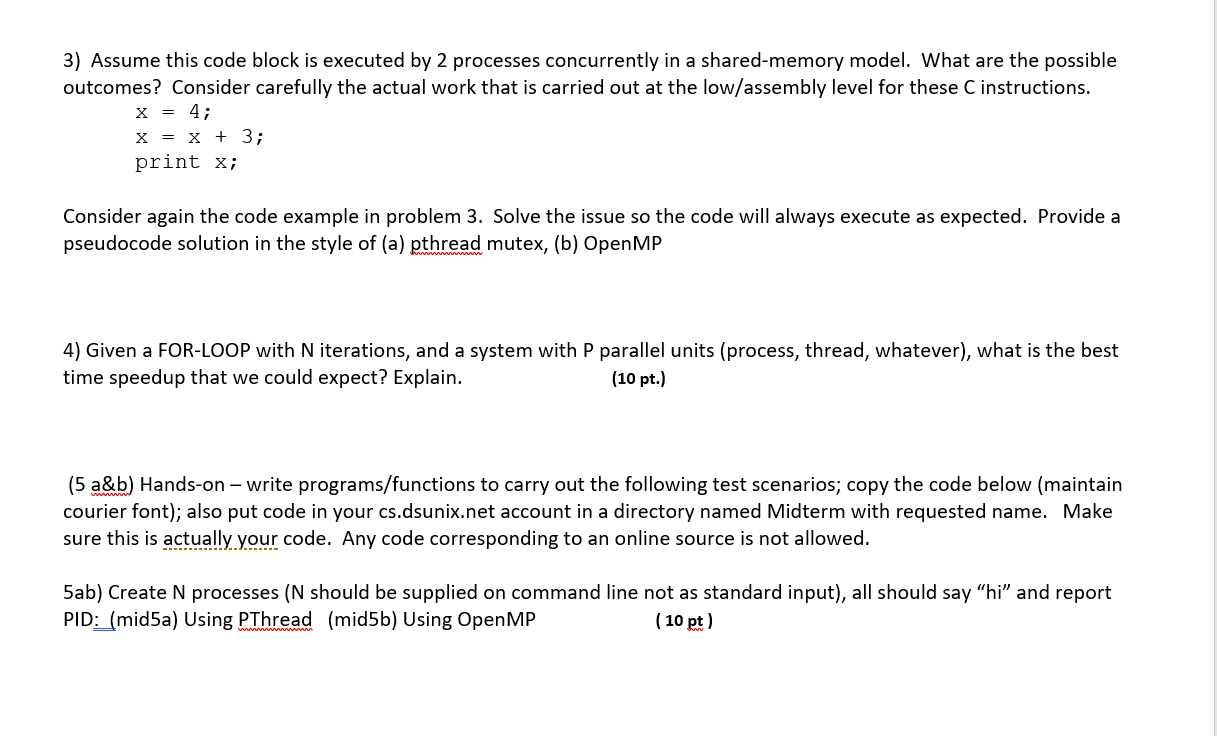
You can also see a couple of crucial functions for dealing with threads. Here the headers for the pthread library are included via pthread.h. Pthread_create ( &thread_id, NULL, thread_callback, &arg ) Printf ( "Inside the thread: %d\n", * ( int * )arg ) Emscripten provides an API-compatible implementation of the pthread library built atop Web Workers, shared memory and atomics, so that the same code can work on the web without changes. In C, particularly on Unix-like systems, the common way to use threads is via POSIX Threads provided by the pthread library.
#Pthread c shared memory how to#
Now let's take a look at how to build a multithreaded version of the WebAssembly module. …now use `module` as you normally would : import ( './module-without-threads.js' ) By default WebAssembly.Memory is a wrapper around an ArrayBuffer-a raw byte buffer that can be accessed only by a single thread. WebAssembly memory is represented by a WebAssembly.Memory object in the JavaScript API. Web Workers have been around for over a decade now, are widely supported, and don't require any special flags. This establishes communication and allows all those threads to run the same WebAssembly code on the same shared memory without going through JavaScript again. Each thread loads a JavaScript glue, and then the main thread uses Worker#postMessage method to share the compiled WebAssembly.Module as well as a shared WebAssembly.Memory (see below) with those other threads. WebAssembly threads use the new Worker constructor to create new underlying threads.

Web Workers #įirst component is the regular Workers you know and love from JavaScript. WebAssembly threads is not a separate feature, but a combination of several components that allows WebAssembly apps to use traditional multithreading paradigms on the web. In this article you will learn how to use WebAssembly threads to bring multithreaded applications written in languages like C, C++, and Rust to the web. It allows you to either run parts of your code in parallel on separate cores, or the same code over independent parts of the input data, scaling it to as many cores as the user has and significantly reducing the overall execution time. WebAssembly threads support is one of the most important performance additions to WebAssembly.
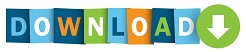